#kryptos #cia #turing #muko
A couple of months ago I decided not to touch K4 until the next clue is given. (Un-)fortunately I did not keep my word.
I decided that I would like to learn something new. This time: Python. Great language. I also decided to try to write something that I have not written yet. I decided to test K4 possiblities of words from another perspective.
What are K4 possiblities of words? Basically these are words that are possible to get from K4 decryption using known encryption algorithm and unknown masking technique.
Until now I tried to find some patterns using method based on:
- select long_word from K4 possibilities
- try to find some pattern between long_word and BC (comparing decrypted and encrypted text)
This time I thought that I will use Python instead of Java and I will try something else.
I tried something like:
import libraries:
from collections import Counter
from pandas import DataFrame
read source data:
possibilitiesFile = ".\\k4_possibilities_of_words_on_positions_from_amartia.txt"
enc = 'utf-8' srcFile = ".\\cryptography_book_dump.txt" with open(srcFile, 'r', encoding = enc) as myfile: raw_data = [myfile.read()]
clean data from the book and remove short words:
whitelist = set('abcdefghijklmnopqrstuvwxyz ABCDEFGHIJKLMNOPQRSTUVWXYZ') clean_data = [ ' '.join(w for w in ''.join(filter(whitelist.__contains__, msg)).lower().split() if len(w)>3) for msg in raw_data]
read data from K4 possibilities:
with open(possibilitiesFile, 'r', encoding = enc) as myfile: raw_possibilities = myfile.readlines() clean_possibilities = [line.rstrip().split(';') for line in raw_possibilities]
and prepare data frames:
df = DataFrame.from_records(clean_possibilities, columns=['Position', 'Word']) mask = (df['Word'].str.len() >= 4) df = df.loc[mask]
and now the idea: lets compare bigrams from the book and K4 possiblities:
bigrams = [bigram for line in clean_data for bigram in zip(line.split(" ")[:-1], line.split(" ")[1:])] counter_bigrams = Counter(bigrams)
lets create bigrams from K4 possibilities:
df['nextPosition'] = df.apply(lambda row: int(row.Position) + len(row.Word), axis=1) df['Position'] = df.apply(lambda row: int(row.Position), axis=1) joined = df.merge(df, left_on=['nextPosition'], right_on=['Position']) tuples = joined[['Word_x', 'Word_y']].values.tolist() new_bigrams = [(tuple[0].lower(), tuple[1].lower()) for tuple in tuples]
now lets try if there is something interesting
selected = [bigram for bigram in new_bigrams if counter_bigrams[bigram] > 0 and len(bigram[0] + bigram[1]) > 9 and (len(bigram[0]) > 5 or len(bigram[1]) > 5)] common = ['with', 'like', 'this'] selected2 = [bigram for bigram in selected if bigram[0] not in common and bigram[1] not in common] print(selected[0])
As I mentioned before, I promised myself not to touch K4 until the next clue is given. I did not keep my word.
print(selected[0])
('turing', 'alan')
and now the horror begins again. I thought I am free of this curse.
Nevertheless feel free to read first part of the book (for free: Muko and the Secret – Part I – free ebook). You could also support the foundation mentioned in the introduction.
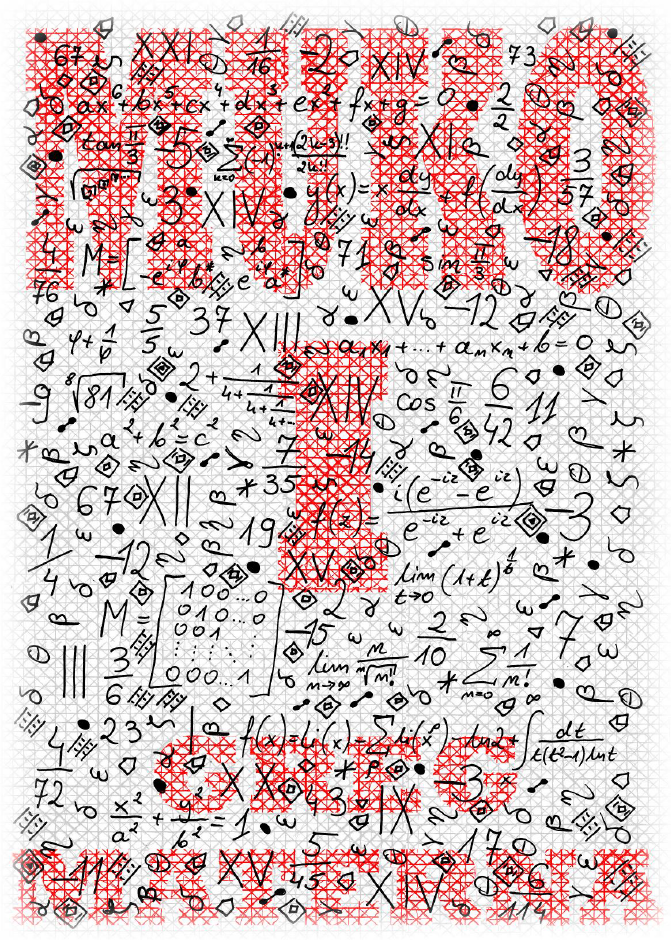
I also encourage you to take a look on the book teaser on youtube: Muko and the Secret – Part I – book teaser
If you liked it – you could also get the second part – (Muko and the Path – part II)
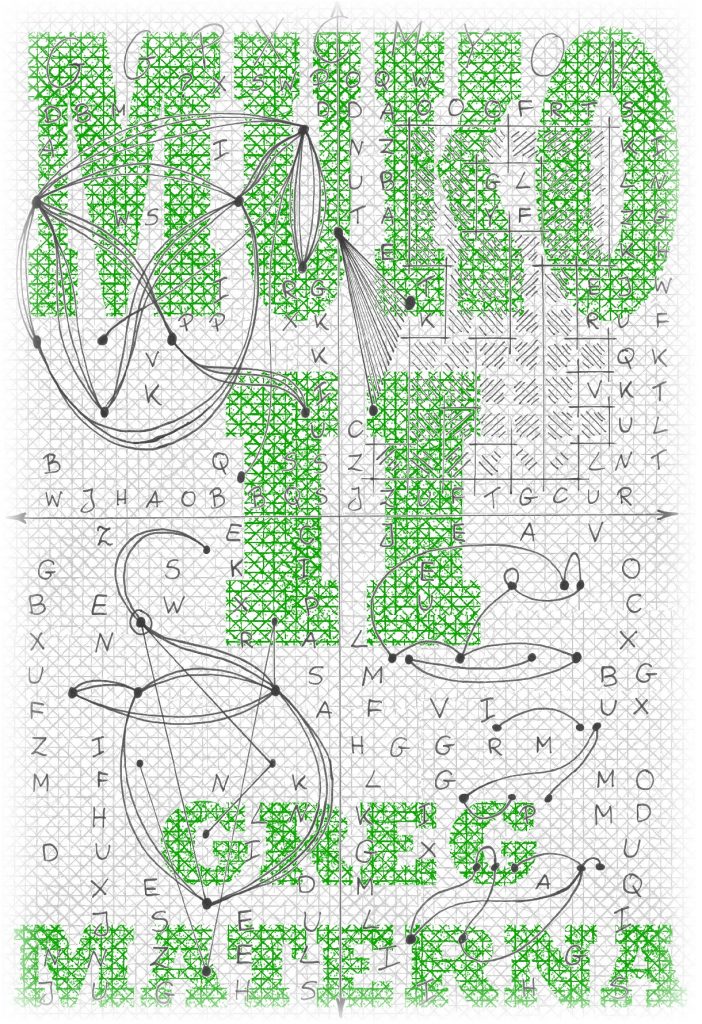